- Introduction to Arrays
- Representation of Arrays
- Array Operations:
- Traversal, Insertion,
- Deletion, Searching
- Multi-dimensional
- Arrays (2D, 3D arrays)
Programming Courses
Data Structure & Algorithm
Have you ever thought of Learning Data Structure & Algorithm?
Based on 99 Reviews
- Duration : 1 Month / 3 Months / 6 Months
- Language: English
- Certificate of Completion
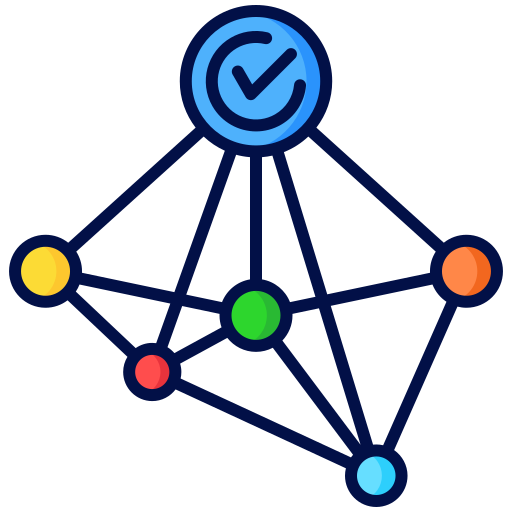
Build a solid foundation in problem-solving and coding efficiency with our Data Structures and Algorithms (DSA) Course, designed for both beginners and experienced programmers. DSA is a critical skill for cracking coding interviews, optimizing software performance, and mastering competitive programming.
This course covers essential data structures like arrays, linked lists, stacks, queues, trees, graphs, and hash tables, as well as algorithms for sorting, searching, recursion, dynamic programming, and greedy techniques. You’ll learn to write clean, efficient, and scalable code while solving real-world problems and participating in coding challenges.
Whether you’re preparing for technical interviews, improving your programming logic, or aiming to excel in competitive coding, this course provides the practical knowledge and confidence to tackle complex problems with ease.
- Introduction to Matrices
- Representation and Operations (addition, multiplication)
- Traversing 2D / 3D arrays
- Introduction to Strings
- String Representation
- String Operations (concatenation, comparison, searching)
- Definition and Operations (Push, Pop, Peek)
- Stack Implementations using Arrays and Linked Lists
- Definition and Operations (Enqueue, Dequeue, Front, Rear)
- Types of Queues: Simple Queue, Circular Queue, Priority Queue, Double-ended Queue
- Introduction to Linked Lists (Singly, Doubly, Circular)
- Operations: Insertion, Deletion, Traversing
- Reversing a Linked List,
- Detecting and Removing Loops
- Introduction to Trees
- Tree Terminology: Root, Leaf, Parent, Child, Depth, Height
- Binary Trees and Traversals: Inorder, Preorder, Postorder
- Binary Tree Operations (Insertion, Deletion, Searching)
- Balanced Trees: AVL Trees, Red-Black Trees
- Properties of Binary Trees
- Binary Tree Traversals (Recursive and Iterative Approaches)
- Insertion, Deletion, Searching
- Introduction to BST
- BST Operations: Search, Insert, Delete
- Balanced BST: AVL Tree, Red-Black Tree
- Introduction to Heaps:
- Max-Heap and Min-Heap
- Heap Operations: Insert, Delete, Heapify
- Heap Sort Algorithm
- Priority Queues
- Introduction to Graphs
- Graph Representations: Adjacency Matrix, Adjacency List
- Types of Graphs: Directed, Undirected, Weighted,
- Unweighted
Graph Traversals: BFS (Breadth-First Search), DFS (Depth-First Search)
- Time Complexity: Big-O Notation, Best, Worst, Average Case
- Space Complexity: Analyzing Space
- Usage of Algorithms
- P vs NP Problem
- NP-Complete and NP-Hard Problems
- Approximation Algorithms
- Linear Search
- Binary Search (Iterative and Recursive)
- Exponential Search
- Interpolation Search
- Introduction to Sorting Algorithms
- Elementary Sorting Algorithms: Bubble Sort, Selection Sort, Insertion Sort
- Efficient Sorting Algorithms: Merge Sort, Quick Sort, Heap Sort
- Non-Comparative Sorting: Counting Sort, Radix Sort
- Introduction to Dynamic Programming
- Memoization vs Tabulation
- Classic DP Problems: Fibonacci, Knapsack Problem, Longest Common Subsequence
- Optimal Substructure and Overlapping Subproblems
- Applications of DP: Coin Change, Matrix Chain Multiplication
- Introduction to Greedy Algorithms
- Greedy Choice Property and Optimal Substructure
- Classic Greedy Problems: Activity Selection, Huffman Coding, Fractional Knapsack
- Introduction to Binary Search
- Binary Search on Sorted Arrays
- Variations: Binary Search on Rotated Arrays, Searching in Infinite Arrays
- Introduction to Backtracking Algorithms
- Backtracking Problems: N-Queens, Sudoku Solver, Rat in a Maze
- Subset Sum Problem
- Applications in Puzzles and Combinatorial Problems
- Introduction to Recursion
- Base Case and Recursive Case
- Recursive Functions: Factorial, Fibonacci Sequence, Tower of Hanoi
- Recursion vs Iteration: Advantages and Disadvantages
- Introduction to Divide and Conquer Algorithms
- Problem Solving with Divide and Conquer: Merge Sort, Quick Sort, Binary Search
- Strassen’s Matrix Multiplication Algorithm
- Introduction to Hashing and Hash Functions
- Hash Table Implementations
- Collision Resolution Techniques: Separate Chaining, Open Addressing
- Applications of Hashing: Caching, Hash Maps, Hash Sets
This Course Include
- Language: English
- Duration : 1 Month / 3 Months / 6 Months
- Learning Mode: At Center ( Only Offline Classes )
- Jurisdiction: Nationwide
- Certificate of Completion
Eligibility Criteria
- Learner should preferably a std. 10th Pass student (Not Compulsory)
- It is desirable that Learner should have done MS-CIT Course (Not Compulsory)
Premium Course
Register For This Course
About Ms. Anjali Arora
Enroll in EduNova’s Flutter App Development Course and master the art of building stunning, high-performance mobile apps for both iOS and Android. Our expert instructors will walk you through each step of the development process, from installation and setup to debugging and deployment. You’ll gain essential programming skills, including Dart fundamentals, such as `const`, `static`, and `final` keywords, as well as Object-Oriented Programming (OOP) in Dart.
Based on 99 Reviews
Ready to take your team to the next level?
Contact us today to learn more about our Corporate Training programs and discover how we can help your organization thrive.
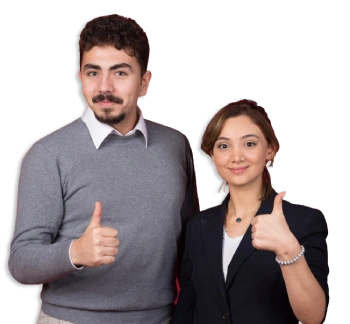
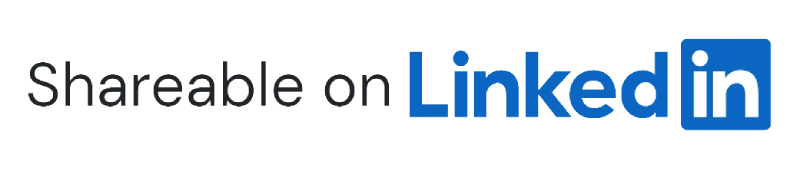
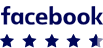
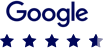
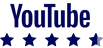
- IITM Pravartak certified Python certification.
- Certificates are globally recognized & they upgrade your programming profile.
- Certificates are generated after the completion of course.
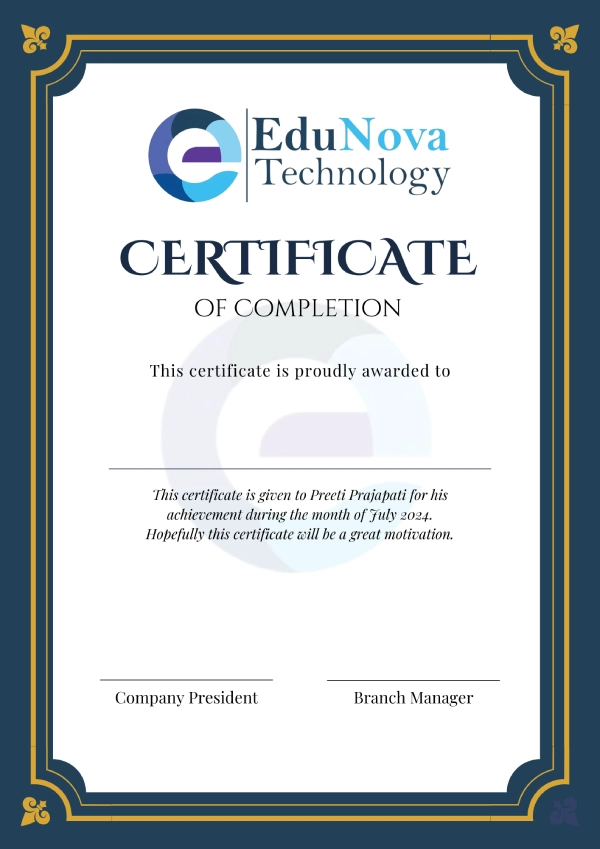
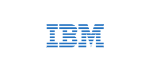
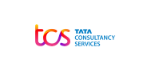
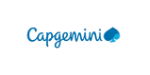
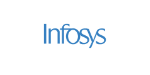
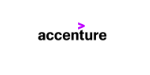
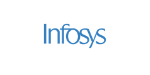

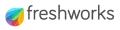
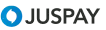



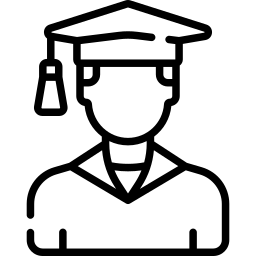
Students/ Job Seekers
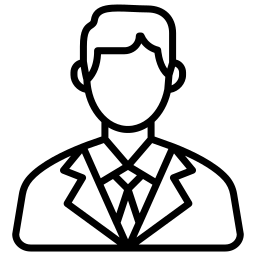
Business
Owners
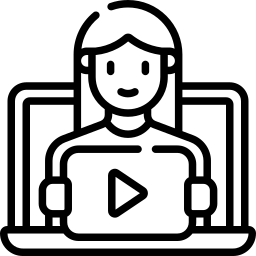
Influencers/ Youtubers
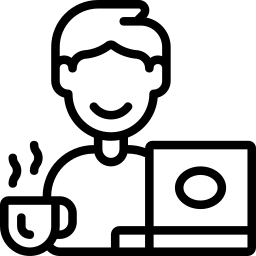
Work as Freelancers
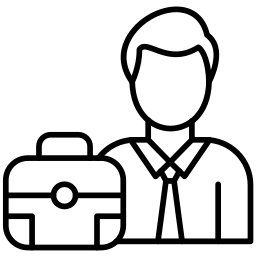
Working Professionals
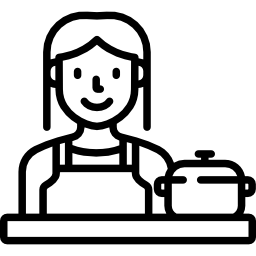
Women / Housewives
They are unique because of their unique approach i.e; Placement, training then Hike. Firstly, they get students placed then give 8 Months of training in any Certification of your choice and at least a 70% hike after 10 months.
Click on Side menu- Choose Courses- Select the course you want to see- Course Detail page will open- click on Start Learning on top- now you can Enrol for the course.
Yes. We offer certifications upon course completion.
Python
Data science
Artificial Intelligence
Digital marketing
Cyber Security
Data Analytics
Quantum computing/ Cloud computing
Android App development
Web development
